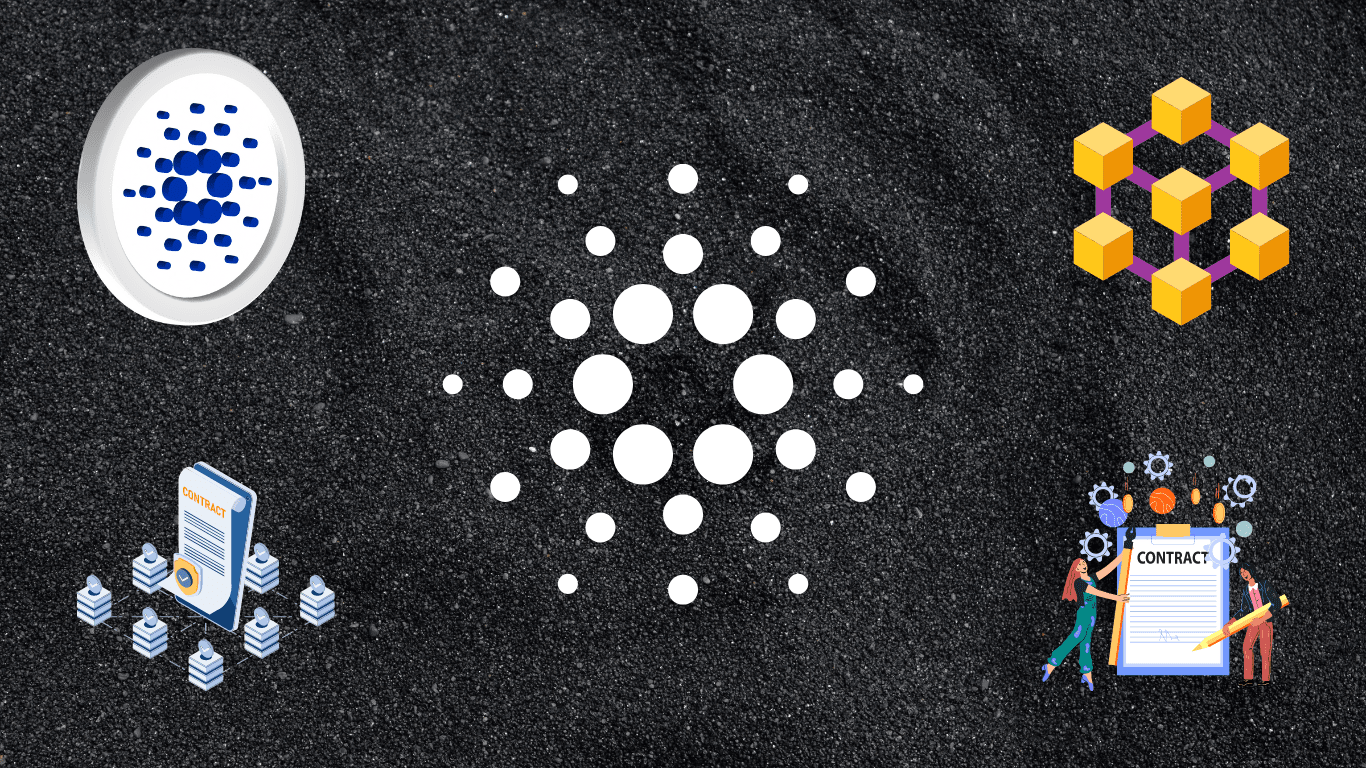
how To create a smart contract on the Cardano blockchain, you will need to use a programming language that is compatible with the Cardano Virtual Machine (CVM). Cardano smart contracts programming language is Plutus, which is a functional programming language specifically designed for use on the Cardano blockchain.
Here is a general outline of the steps you can follow to create a smart contract on Cardano:
- Install the necessary tools and dependencies:
- Install the Cardano wallet, which will allow you to manage your assets and interact with the Cardano blockchain.
- Install the Cardano development environment, which includes the Plutus Platform and other tools that you will need to develop and deploy smart contracts.
- Learn Plutus:
- Familiarize yourself with the Plutus programming language by reading the documentation and working through some tutorial exercises.
- Write and test your smart contract code using the Plutus Platform, which provides a sandbox environment for developing and testing Plutus code.
- Deploy your smart contract:
- Once you have written and tested your smart contract code, you can use the Cardano wallet to deploy it to the Cardano blockchain. This will make it available for use by other users.
- Interact with your smart contract:
- You can use the Cardano wallet or other tools to interact with your smart contract, such as calling its functions or querying its state. You can also use these tools to monitor the performance of your smart contract and troubleshoot any issues that may arise.
It is recommended that you have some experience with programming and blockchain technology before attempting to create a smart contract on Cardano. If you are new to these topics, you may want to start by learning the basics and gaining some practical experience before diving into the specifics of creating smart contracts on Cardano.
Install the necessary tools and dependencies
Yes, that is correct. In order to create a smart contract on the Cardano blockchain, you will need to install the necessary tools and dependencies.
The first step is to install the Cardano wallet, which is a tool that allows you to manage your assets and interact with the Cardano blockchain. The Cardano wallet is available for various platforms, including Windows, Mac, and Linux, and can be downloaded from the Cardano website or through a package manager such as Homebrew or Chocolatey.
Once you have installed the Cardano wallet, you will need to install the Cardano development environment. This includes the Plutus Platform and other tools that you will need to develop and deploy smart contracts. The Cardano development environment is also available for various platforms and can be downloaded from the Cardano website or through a package manager.
After installing the necessary tools and dependencies, you can then proceed to learn the Plutus programming language and write and test your smart contract code using the Plutus Platform. Once you are satisfied with your code, you can use the Cardano wallet to deploy it to the Cardano blockchain and interact with it using the wallet or other tools. Regenerate response
Learn Plutus:
Yes, that is correct. To create a smart contract on the Cardano blockchain, you will need to learn the Plutus programming language and write and test your smart contract code using the Plutus Platform.
To familiarize yourself with Plutus, you can start by reading the documentation and working through some tutorial exercises. This will help you understand the syntax and features of the language, as well as the overall structure and behavior of smart contracts in the Cardano ecosystem.
Once you have a basic understanding of Plutus, you can start writing your own smart contract code. The Plutus Platform provides a sandbox environment for developing and testing Plutus code, which allows you to write, compile, and test your smart contract code without having to deploy it to the actual Cardano blockchain. This can be a useful tool for debugging and verifying the correctness of your code before you deploy it.
Once you have written and tested your smart contract code using the Plutus Platform, you can then use the Cardano wallet to deploy it to the Cardano blockchain. This will make it available for use by other users, and you can then interact with your smart contract using the Cardano wallet or other tools.
Deploy your smart contract:
Yes, that is correct. Once you have written and tested your smart contract code using the Plutus Platform, you can use the Cardano wallet to deploy it to the Cardano blockchain. This will make your smart contract available for use by other users.
To deploy your smart contract to the Cardano blockchain, you will need to do the following:
- Use the Plutus Platform to compile your smart contract code into an executable form that can be run on the Cardano Virtual Machine (CVM).
- Use the Cardano wallet to create a transaction that includes your compiled smart contract code and any necessary data or arguments.
- Sign and broadcast the transaction using the Cardano wallet. This will send your transaction to the Cardano network, where it will be validated and included in a block by a network of decentralized “miners”.
- Once your transaction has been included in a block and added to the blockchain, your smart contract will be deployed and available for use.
It is important to note that deploying a smart contract to the Cardano blockchain is a permanent action. Once your smart contract is deployed, it cannot be modified or deleted, and it will continue to exist and be available for use until the end of the Cardano blockchain. Therefore, it is important to thoroughly test and verify your smart contract code before deploying it to the blockchain.
Interact with your smart contract:
Yes, that is correct. Once you have deployed your smart contract to the Cardano blockchain, you can use the Cardano wallet or other tools to interact with it. This allows you to execute the functions and features of your smart contract, as well as query its state and data.
To interact with your smart contract, you will need to do the following:
- Use the Cardano wallet or other tools to create a transaction that includes a call to one of the functions or features of your smart contract.
- Sign and broadcast the transaction using the Cardano wallet or other tools. This will send your transaction to the Cardano network, where it will be validated and included in a block by a network of decentralized “miners”.
- Once your transaction has been included in a block and added to the blockchain, the function or feature of your smart contract will be executed and the results will be recorded on the blockchain.
You can use these tools to interact with your smart contract in various ways, such as calling its functions, querying its state, or monitoring its performance. You can also use these tools to troubleshoot any issues that may arise while interacting with your smart contract.
It is important to note that interacting with a smart contract on the Cardano blockchain involves sending transactions to the network and waiting for them to be validated and included in a block. This process can take some time, and the results of your interactions with the smart contract may not be immediately available. Therefore, it is important to plan and design your smart contract interactions carefully to ensure that they are efficient and effective.
Cardano smart contract GitHub
You can find a variety of resources related to Cardano smart contracts on GitHub, including code examples, tutorials, and tools.
Here are a few examples of repositories that you might find useful:
- The official Plutus repository, which contains documentation and resources for the Plutus programming language, including a tutorial and examples of Plutus code: https://github.com/input-output-hk/plutus
- The Plutus Playground, which is an online platform for writing and testing Plutus code: https://github.com/input-output-hk/plutus-playground
- The Cardano development environment, which includes the Plutus Platform and other tools for developing and deploying smart contracts on Cardano: https://github.com/input-output-hk/cardano-devel
- The Cardano wallet, which is a tool for managing assets and interacting with the Cardano blockchain: https://github.com/input-output-hk/cardano-wallet
You can also find a variety of third-party resources related to Cardano smart contracts on GitHub, including code examples, libraries, and tools. These resources may be created and maintained by community members and are not affiliated with the official Cardano project.
It is important to carefully evaluate any resources that you find on GitHub or elsewhere before using them, as they may not always be reliable or up-to-date. It is also a good idea to familiarize yourself with the basics of smart contract development and the Cardano ecosystem before attempting to use these resources.
Cardano smart contracts example
Here is a simple example of a smart contract written in Plutus, the programming language used to create smart contracts on the Cardano blockchain:
module Main where
import Prelude hiding (either)
import Data.Either
import Ledger
import Wallet
import qualified Data.Map as Map
type State = Map.Map Address Integer
data Action
= Deposit Address Integer
| Withdraw Address Integer
| Query Address
deriving (Show, Eq)
deposit
:: Address
-> Integer
-> State
-> State
deposit addr amount state =
Map.insert addr newBalance state
where
newBalance =
maybe amount (amount +) (Map.lookup addr state)
withdraw
:: Address
-> Integer
-> State
-> Either String State
withdraw addr amount state =
if balance < amount
then Left "Insufficient balance"
else Right newState
where
balance =
maybe 0 id (Map.lookup addr state)
newState =
Map.insert addr (balance - amount) state
query
:: Address
-> State
-> Integer
query addr state =
maybe 0 id (Map.lookup addr state)
apply
:: Action
-> State
-> Either String State
apply action state =
case action of
Deposit addr amount ->
Right (deposit addr amount state)
Withdraw addr amount ->
withdraw addr amount state
Query addr ->
Right (query addr state)
main :: IO ()
main =
do
putText "Enter an action (Deposit, Withdraw, or Query):"
action <- getLine
case action of
"Deposit" ->
do
putText "Enter an address:"
addr <- getLine
putText "Enter an amount:"
amount <- getText
let result =
apply (Deposit addr amount) Map.empty
case result of
Right newState ->
putText "Success"
Left err ->
putText err
"Withdraw" ->
do
putText "Enter an address:"
addr <- getLine
putText "Enter an amount:"
amount <- getText
let result =
apply (Withdraw addr amount) Map.empty
case result of
Right newState ->
putText "Success"
Left err ->
putText err
"Query" ->
do
putText "Enter an address:"
addr <- getLine
let result = apply (Query addr) Map.empty
case result of
Right balance ->
putText (show balance)
Left err ->
putText err
_ ->
putText "Invalid action"
This smart contract provides a simple “wallet” contract that allows users to deposit and withdraw funds, as well as query their balance. It includes three main functions: deposit
, withdraw
, and query
. The deposit
the function allows users to add funds to their wallets, while the withdraw
function allows them to remove funds. The query
function allows users to check their current balance.
To deploy and interact with this smart contract on the Cardano blockchain, you will need to use the Plutus Platform and the Cardano wallet,